7 DGE model building with variancePartition
Instructor: Daianna González-Padilla
After having processed RNA-seq data and assessed the quality and the variability of the samples the next step for DGE is to explore the variance in the expression of the genes themselves according to sample groups, or in other words, to quantify the contribution of the multiple sample variables in the gene expression variation.
To determine which variables are the major drivers of expression variability, and importantly to define if the technical variability of RNA-seq data is low enough to study the condition of interest, we can implement an analysis of variance partition. variancePartition is a package that decomposes for each gene the expression variation into fractions of variance explained (FVE) by the sample variables in the experimental design of high-throughput genomics studies [1].
In order to exemplify how to implement this analysis and the type of conclusions that can be drawn from it, we’ll use bulk RNA-seq data from the smokingMouse package.
## Load the container package for this type of data
library("SummarizedExperiment")
## Connect to ExperimentHub
library("ExperimentHub")
eh <- ExperimentHub::ExperimentHub()
## Load the datasets of the package
myfiles <- query(eh, "smokingMouse")
## Download the mouse gene data
rse_gene <- myfiles[["EH8313"]]
## Keep samples from nicotine experiment and pups only
rse_gene_nic <- rse_gene[
,
which(rse_gene$Expt == "Nicotine" & rse_gene$Age == "Pup")
]
## Use expressed genes only (i.e. that passed the filtering step)
rse_gene_filt <- rse_gene_nic[
rowData(rse_gene_nic)$retained_after_feature_filtering,
]
## Keep samples that passed QC and manual sample filtering steps (all passed)
rse_gene_filt <- rse_gene_filt[
,
rse_gene_filt$retained_after_QC_sample_filtering &
rse_gene_filt$retained_after_manual_sample_filtering
]
7.1 Canonical Correlation Analysis
Prior to the variance partition analysis, evaluating the correlation between sample variables is crucial because highly correlated variables can produce unstable estimates of the variance fractions and impede the identification of the variables that really contribute to the expression variation. There are at least two problems with correlated variables:
- If two variables are highly correlated we could incorrectly determine that one of them contributes to gene expression changes when it was actually not explanatory but just correlated with a real contributory variable.
- The part of variance explained by a biologically relevant variable can be reduced by the apparent contributions of correlated variables, if for example, they contain very similar information (i.e. are redundant variables).
Additionally, the analysis is better performed with simpler models, specially when we have a limited number of samples in the study.
Hence, to remove such variables we must first identify them. We will perform a Canonical Correlation Analysis (CCA) with canCorPairs()
that assesses the degree to which the variables co-vary and contain the same information. With CCA, linear combinations that maximize the correlation between variable sets are estimated. CCA is just like a normal correlation analysis between 2 vectors but it can accommodate matrices as well (variable sets). Note that CCA returns correlations values between 0 and 1 [2].
library("variancePartition")
library("pheatmap")
## Plot heatmap of correlations
## Define all variables to examine; remove those with single values
formula <- ~ Group + Sex + plate + flowcell + mitoRate + overallMapRate + totalAssignedGene + rRNA_rate + sum + detected + ERCCsumLogErr
## Measure correlations
CCA <- canCorPairs(formula, colData(rse_gene_filt))
## Heatmap
pheatmap(
CCA, ## data
color = hcl.colors(50, "YlOrRd", rev = TRUE), ## color scale
fontsize = 8, ## text size
border_color = "black", ## border color for heatmap cells
cellwidth = unit(0.4, "cm"), ## height of cells
cellheight = unit(0.4, "cm") ## width of cells
)
⚠️ Very important: always inspect if there are any correlated variables with the one of interest in your study! This is extremely important as correlated variables could represent confounding factors and/or hinder the detection of significant DE events, thus yielding to misleading results.
Importantly, Group
is not highly correlated with any other variable in this study, but overallMapRate
is correlated with rRNA_rate
, library preparation plate
, and the sequencing flowcell
; sum
(library size) and detected
(number of expressed genes) are also correlated. For a detailed definition of these variables check here.
📝 Exercise 1: Run a CCA analysis and determine which pairs of variables in your dataset are correlated. Is there any correlated variable with your variable of interest?
Depending on your results there’s sometimes convenient to dig a little deeper into the relationship between correlated variables and to analyze these metrics among our control and experimental samples. Let’s work on that!
library("ggplot2")
library("cowplot")
## Boxplots/Scatterplots/Barplots for each pair of correlated variables
corr_plots <- function(sample_var1, sample_var2, sample_color) {
## Define sample colors by variable
colors <- list(
"Group" = c("Control" = "brown2", "Experimental" = "deepskyblue3"),
"Sex" = c("F" = "hotpink1", "M" = "dodgerblue"),
"plate" = c("Plate1" = "darkorange", "Plate2" = "lightskyblue", "Plate3" = "deeppink1"),
"flowcell" = c(
"HKCG7DSXX" = "chartreuse2", "HKCMHDSXX" = "magenta",
"HKCNKDSXX" = "turquoise3", "HKCTMDSXX" = "tomato"
)
)
data <- colData(rse_gene_filt)
## a) Barplots for categorical variable vs categorical variable
if (class(data[, sample_var1]) == "character" & class(data[, sample_var2]) == "character") {
## y-axis label
y_label <- paste("Number of samples from each ", sample_var2, sep = "")
## Stacked barplot with counts for 2nd variable
plot <- ggplot(data = as.data.frame(data), aes(
x = !!rlang::sym(sample_var1),
fill = !!rlang::sym(sample_var2)
)) +
geom_bar(position = "stack") +
## Colors by 2nd variable
scale_fill_manual(values = colors[[sample_var2]]) +
## Show sample counts on stacked bars
geom_text(aes(label = after_stat(count)),
stat = "count",
position = position_stack(vjust = 0.5), colour = "gray20", size = 3
) +
theme_bw() +
labs(
subtitle = paste0("Corr: ", signif(CCA[sample_var1, sample_var2], digits = 3)),
y = y_label
) +
theme(
axis.title = element_text(size = (7)),
axis.text = element_text(size = (6)),
plot.subtitle = element_text(size = 7, color = "gray40"),
legend.text = element_text(size = 6),
legend.title = element_text(size = 7)
)
}
## b) Boxplots for categorical variable vs continuous variable
else if (class(data[, sample_var1]) == "character" & class(data[, sample_var2]) == "numeric") {
plot <- ggplot(data = as.data.frame(data), mapping = aes(
x = !!rlang::sym(sample_var1),
y = !!rlang::sym(sample_var2),
color = !!rlang::sym(sample_var1)
)) +
geom_boxplot(size = 0.25, width = 0.32, color = "black", outlier.color = NA) +
geom_jitter(width = 0.15, alpha = 1, size = 1.5) +
stat_smooth(method = "lm", geom = "line", alpha = 0.6, size = 0.4, span = 0.3, aes(group = 1), color = "orangered3") +
scale_color_manual(values = colors[[sample_var1]]) +
theme_bw() +
guides(color = "none") +
labs(
subtitle = paste0("Corr: ", signif(CCA[sample_var1, sample_var2], digits = 3)), y = gsub("_", " ", sample_var2),
x = sample_var1
) +
theme(
axis.title = element_text(size = (7)),
axis.text = element_text(size = (6)),
plot.subtitle = element_text(size = 7, color = "gray40"),
legend.text = element_text(size = 6),
legend.title = element_text(size = 7)
)
}
## c) Scatterplots for continuous variable vs continuous variable
else if (class(data[, sample_var1]) == "numeric" & class(data[, sample_var2]) == "numeric") {
plot <- ggplot(as.data.frame(data), aes(
x = !!rlang::sym(sample_var1),
y = !!rlang::sym(sample_var2),
color = !!rlang::sym(sample_color)
)) +
geom_point(size = 2) +
stat_smooth(method = "lm", geom = "line", alpha = 0.6, size = 0.6, span = 0.25, color = "orangered3") +
## Color by sample_color variable
scale_color_manual(name = sample_color, values = colors[[sample_color]]) +
theme_bw() +
labs(
subtitle = paste0("Corr: ", signif(CCA[sample_var1, sample_var2], digits = 3)),
y = gsub("_", " ", sample_var2),
x = gsub("_", " ", sample_var1)
) +
theme(
axis.title = element_text(size = (7)),
axis.text = element_text(size = (6)),
plot.subtitle = element_text(size = 7, color = "gray40"),
legend.text = element_text(size = 6),
legend.title = element_text(size = 7)
)
}
return(plot)
}
As shown below, Group
and plate
are moderately correlated given that 14 of the 23 (60.8%) control samples and 11 of the 19 (57.9%) exposed samples were in the first and second plate for library preparation, respectively.
## Correlation plot for Group and plate
p <- corr_plots("Group", "plate", NULL)
p + theme(plot.margin = unit(c(1, 5.5, 1, 5.5), "cm"))
We can also observe that even though QC metrics such as overallMapRate
and rRNA_rate
are correlated, there’s no distinction between control and exposed samples for these variables.
## Correlation plot for overallMapRate and rRNA_rate
p <- corr_plots("overallMapRate", "rRNA_rate", "Group")
p + theme(plot.margin = unit(c(2, 3.5, 2, 3.5), "cm"))
Moreover, the correlation between overallMapRate
and the library preparation plate
is mainly given by the plate 1 samples that have lower rates, similar to what occurs with the samples from the first flowcell.
## Correlation plot for overallMapRate and plate
p <- corr_plots("plate", "overallMapRate", NULL)
p + theme(plot.margin = unit(c(2, 5, 2, 5), "cm"))
## Correlation plot for overallMapRate and flowcell
p <- corr_plots("flowcell", "overallMapRate", NULL)
p + theme(plot.margin = unit(c(2, 5, 2, 5), "cm"))
Interestingly, control samples seem to present more expressed genes than exposed samples for a given library size,
however none of these variables is correlated with Group
.
## Correlation plots for sum and detected
p <- corr_plots("sum", "detected", "Group")
p + theme(plot.margin = unit(c(2, 3.5, 2, 3.5), "cm"))
❓ Now look at the following plot. Why is it important that experimental and control samples are distributed throughout all sequencing flowcells?
p <- corr_plots("Group", "flowcell", NULL)
plots <- plot_grid(p)
plots + theme(plot.margin = unit(c(0.5, 5, 0.5, 5), "cm"))
Hint: What would happen if all experimental samples were in one flowcell and all controls in another?
After identifying which variables are correlated and exploring the metrics of control and experimental samples the next is to determine which variable from each pair of correlated variables should be discarded and which one included in the models. How do we discern which ones to keep? As recommended in the variancePartition user’s guide [2], initially we can fit a linear model to the expression data of each gene taking all sample variables and then investigate which ones explain higher percentages of variance for many genes. But first let’s review how variancePartition works.
7.2 Fit model and extract fraction of variance explained
Briefly, what variancePartition does is to fit a linear model for each gene separately and to compute the fraction of the total data variance explained by each variable of the study design, as well as by the residuals, using the calcVarPart()
function. These computed fractions of variation explained (FVE) summarize the contribution of each variable and naturally sum to 1 [1].
variancePartition fits two types of models:
- Linear mixed model (LMM) where all categorical variables are modeled as random effects and all continuous variables are fixed effects. The function
lmer()
from lme4 is used to fit this model.
## Fit LMM specifying the existence of random effects with '(1| )'
fit <- lmer(expr ~ a + b + (1|c), data=data)
- Fixed effects model, which is basically the standard linear model (LM), where all variables are modeled as fixed effects. The function
lm()
is used to fit this model.
In our case, the function will be modeled as a mixed model since we have both effects.
❓ What are random and fixed effects? Categorical variables are usually modeled as random effects, i.e., variables such as flowcell, plate, donor, etc. whose levels are “randomly chosen or selected from a larger population”. These levels are not of interest by themselves but the grouping of the samples by them. Random effects correspond to those variables whose effect on the expression of a gene varies according to its sample groups/levels. On the other hand, continuous variables can be modeled as fixed effects. These are sample-level variables that preserve their impact on the expression of a gene irrespective of the sample.
❓ Why is this effect distinction important? Because when we have clustered data, like gene expression values grouped by sample sex, batch, etc. we are violating the relevant assumption of independence, making an incorrect inference when using a general linear model (GLM). If we have clustered data where the variables’ values have distinct effects on gene expression, we must work with an extension of GLM, i.e. with the linear mixed model (LMM) that contains a mix of both fixed and random effects [3].
Linear mixed model fit
👉🏼 Source code of calcVarPart()
here.
Once we have reviewed what variancePartition computes and how, we can use it to quantify the FVE for each variable.
## Fit a linear mixed model (LMM) that takes continuous variables as fixed effects and categorical variables as random effects
varPartAnalysis <- function(formula) {
## Ignore genes with variance 0
genes_var_zero <- which(apply(assays(rse_gene_filt)$logcounts, 1, var) == 0)
if (length(genes_var_zero) > 0) {
rse_gene_filt <- rse_gene_filt[-genes_var_zero, ]
}
## Loop over each gene to fit the model and extract variance explained by each variable
varPart <- fitExtractVarPartModel(assays(rse_gene_filt)$logcounts, formula, colData(rse_gene_filt))
# Sort variables by median fraction of variance explained (FVE)
vp <- sortCols(varPart)
p <- plotVarPart(vp)
return(list(p, vp))
}
In the following violin plots, we have the % of variance explained in the expression of each gene by each covariate, based on the model with all variables. Of our pairs of correlated variables, rRNA_rate
has the highest median FVE and thus, should be included in the models for DGE, whereas variables correlated with it (overallMapRate
) must be removed. Furthermore, library preparation plate
must be excluded as it is correlated with Group
.
##### Fit model with all variables #####
# sum, detected, and ERCCsumLogErr are not included as they are in very different scales!
formula <- ~ (1 | Group) + (1 | Sex) + (1 | plate) + (1 | flowcell) + mitoRate + overallMapRate +
totalAssignedGene + rRNA_rate
plot <- varPartAnalysis(formula)[[1]]
plot + theme(
plot.margin = unit(c(1, 1, 1, 1), "cm"),
axis.text.x = element_text(size = (7)),
axis.text.y = element_text(size = (7.5))
)
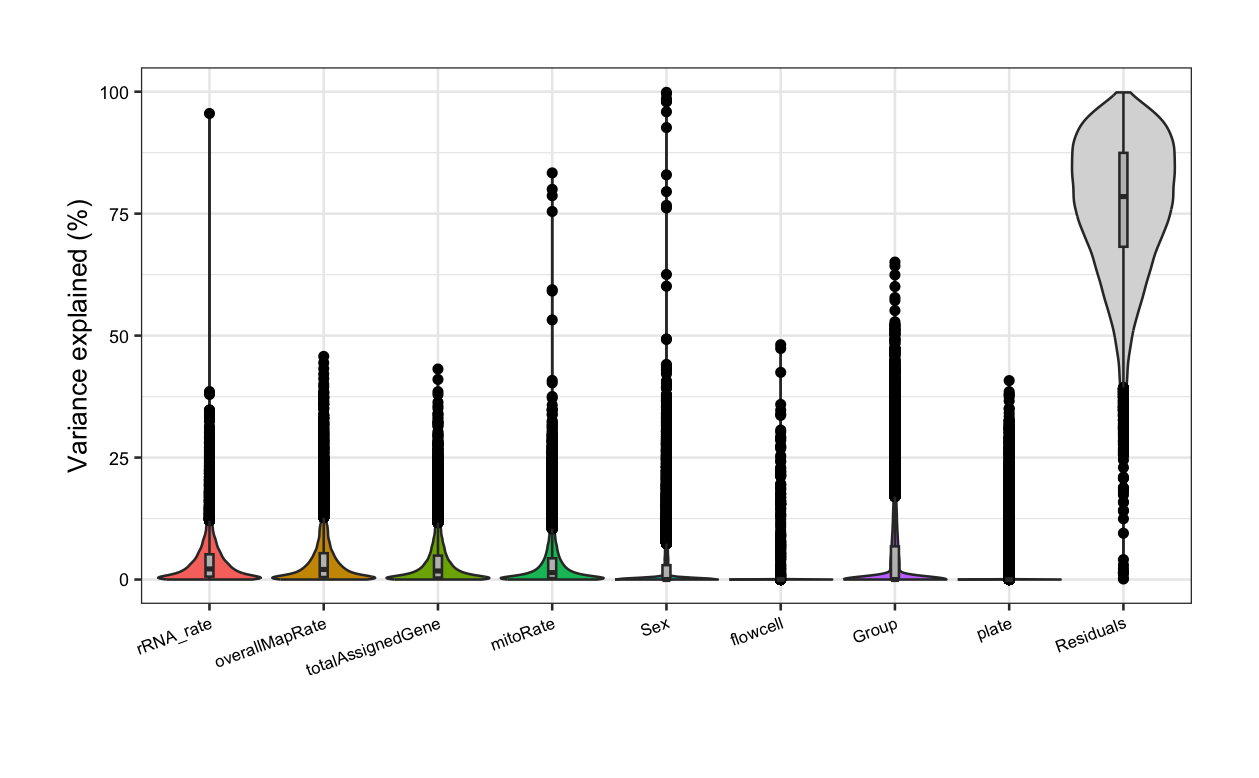
⚠️ Note that some variables such as the library size and the number of detected genes that are in different orders of magnitude to the rest of the QC metrics and categorical variables are not included in this analysis as they can impact the model predictions and the interpretability of the regression results [4]. These variables can be analyzed only after rescaling.
After re-running the analysis without the previous correlated variables, now Group
contribution increases but so does the residual source, i.e., the % of gene expression variance that the model couldn’t explain increases, although the increase is rather low. This occurs because when we remove independent variables to a regression equation, we can explain less of the variance of the dependent variable [3]. That’s the price to pay when dropping variables, but it is convenient when we don’t have many samples for the model to determine variable unique contributions.
##### Fit model without correlated variables #####
## Pup plots without overallMapRate and plate
formula <- ~ (1 | Group) + (1 | Sex) + (1 | flowcell) + mitoRate + overallMapRate + totalAssignedGene
varPart <- varPartAnalysis(formula)
varPart_data <- varPart[[2]]
plot <- varPart[[1]]
plot + theme(
plot.margin = unit(c(1, 1, 1, 1), "cm"),
axis.text.x = element_text(size = (7)),
axis.text.y = element_text(size = (7.5))
)
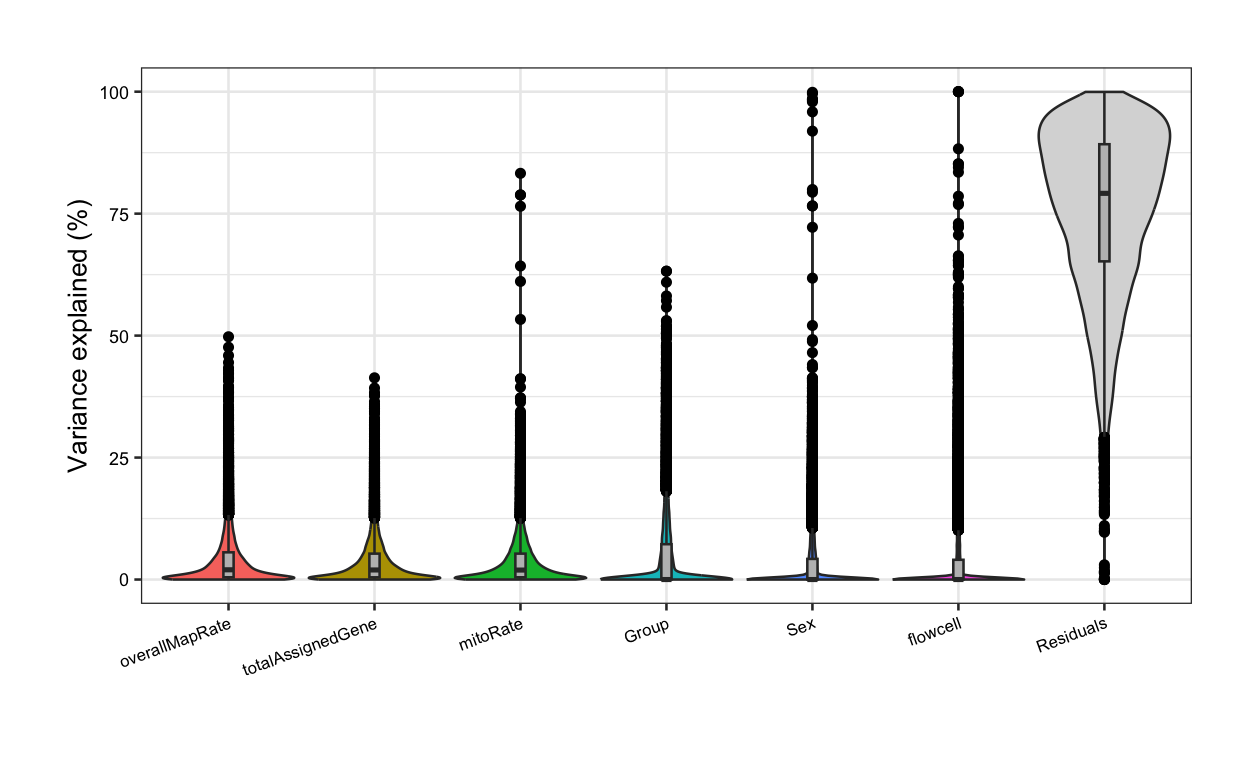
📝 Exercise 2: Perform a variance partition analysis and determine which of your correlated variables have higher contributions in gene expression variance. Based on that, select a set of variables to model gene expression for DGE.
But what does it mean that a variable explains a high percentage of the expression variation of a gene? In the following section will visualize the existing relationships between the gene expression values in the samples and the sample-level variables.
7.3 Examine the expression of most affected genes by each sample variable
In the plots presented below we can appreciate the expression levels across samples of the most affected genes by each variable, i.e., the genes for which the variable explains the highest percentages of variance, plotted against the sample values for the same variable. Observe the strong correlations that exist for the sample variables and the gene expression of such affected genes, which ends up causing these variables to explain high percentages of gene expression variation and which obligate us to adjust for them in the models.
library("rlang")
## Plot of gene expression lognorm counts vs. sample variable
plot_gene_expr <- function(sample_var, gene_id) {
colors <- list(
"Group" = c("Control" = "brown2", "Experimental" = "deepskyblue3"),
"Age" = c("Adult" = "slateblue3", "Pup" = "yellow3"),
"Sex" = c("F" = "hotpink1", "M" = "dodgerblue"),
"Pregnancy" = c("Yes" = "darkorchid3", "No" = "darkolivegreen4"),
"plate" = c("Plate1" = "darkorange", "Plate2" = "lightskyblue", "Plate3" = "deeppink1"),
"flowcell" = c(
"HKCG7DSXX" = "chartreuse2", "HKCMHDSXX" = "magenta", "HKCNKDSXX" = "turquoise3",
"HKCTMDSXX" = "tomato"
)
)
## Lognorm counts of the gene across samples
data <- colData(rse_gene_filt)
data$gene_expr <- assays(rse_gene_filt)$logcounts[gene_id, ]
## Percentage of variance explained by the variable
percentage <- 100 * signif(varPart_data[gene_id, sample_var], digits = 3)
## Boxplots for categorical variables
if (class(data[, sample_var]) == "character") {
plot <- ggplot(data = as.data.frame(data), mapping = aes(
x = !!rlang::sym(sample_var),
y = gene_expr, color = !!rlang::sym(sample_var)
)) +
geom_boxplot(size = 0.25, width = 0.32, color = "black", outlier.color = "#FFFFFFFF") +
geom_jitter(width = 0.15, alpha = 1, size = 1) +
stat_smooth(geom = "line", alpha = 0.6, size = 0.4, span = 0.3, method = "lm", aes(group = 1), color = "orangered3") +
scale_color_manual(values = colors[[sample_var]]) +
theme_bw() +
guides(color = "none") +
labs(
title = gene_id,
subtitle = paste0("Variance explained: ", percentage, "%"),
y = "lognorm counts", x = sample_var
) +
theme(
axis.title = element_text(size = (7)),
axis.text = element_text(size = (6)),
plot.title = element_text(hjust = 0.5, size = 7.5, face = "bold"),
plot.subtitle = element_text(size = 7, color = "gray40"),
legend.text = element_text(size = 6),
legend.title = element_text(size = 7)
)
}
## Scatterplots for continuous variables
else {
colors <- c(
"mitoRate" = "khaki3", "overallMapRate" = "turquoise", "totalAssignedGene" = "plum2", "rRNA_rate" = "orange3",
"sum" = "palegreen3", "detected" = "skyblue2", "ERCCsumLogErr" = "slateblue1"
)
plot <- ggplot(as.data.frame(data), aes(x = eval(parse_expr(sample_var)), y = gene_expr)) +
geom_point(color = colors[[sample_var]], size = 2) +
stat_smooth(geom = "line", alpha = 0.4, size = 0.4, span = 0.25, method = "lm", color = "orangered3") +
theme_bw() +
guides(color = "none") +
labs(
title = gene_id,
subtitle = paste0("Variance explained: ", percentage, "%"),
y = "lognorm counts", x = gsub("_", " ", sample_var)
) +
theme(
plot.margin = unit(c(0.4, 0.1, 0.4, 0.1), "cm"),
axis.title = element_text(size = (7)),
axis.text = element_text(size = (6)),
plot.title = element_text(hjust = 0.5, size = 7.5, face = "bold"),
plot.subtitle = element_text(size = 7, color = "gray40"),
legend.text = element_text(size = 6),
legend.title = element_text(size = 7)
)
}
return(plot)
}
## Function to plot gene expression vs sample variable data for top 3 most affected genes
plot_gene_expr_sample <- function(sample_var) {
## Top 3 genes most affected by sample variable
affected_genes <- rownames(varPart_data[order(varPart_data[, sample_var], decreasing = TRUE), ][1:3, ])
## Plots
plots <- list()
for (i in 1:length(affected_genes)) {
plots[[i]] <- plot_gene_expr(sample_var, affected_genes[i])
}
plot_grid(plots[[1]], plots[[2]], plots[[3]], ncol = 3)
}
## Plots for top affected genes by 'overallMapRate'
plots <- plot_gene_expr_sample("overallMapRate")
plots + theme(plot.margin = unit(c(3, 1, 2, 3), "cm"))
## Plots for top affected genes by 'totalAssignedGene'
plots <- plot_gene_expr_sample("totalAssignedGene")
plots + theme(plot.margin = unit(c(3, 1, 2, 3), "cm"))
## Plots for top affected genes by 'Group'
plots <- plot_gene_expr_sample("Group")
plots + theme(plot.margin = unit(c(3, 1, 2, 3), "cm"))
## Plots for top affected genes by 'Sex' (genes in sexual chrs)
plots <- plot_gene_expr_sample("Sex")
plots + theme(plot.margin = unit(c(3, 1, 2, 3), "cm"))
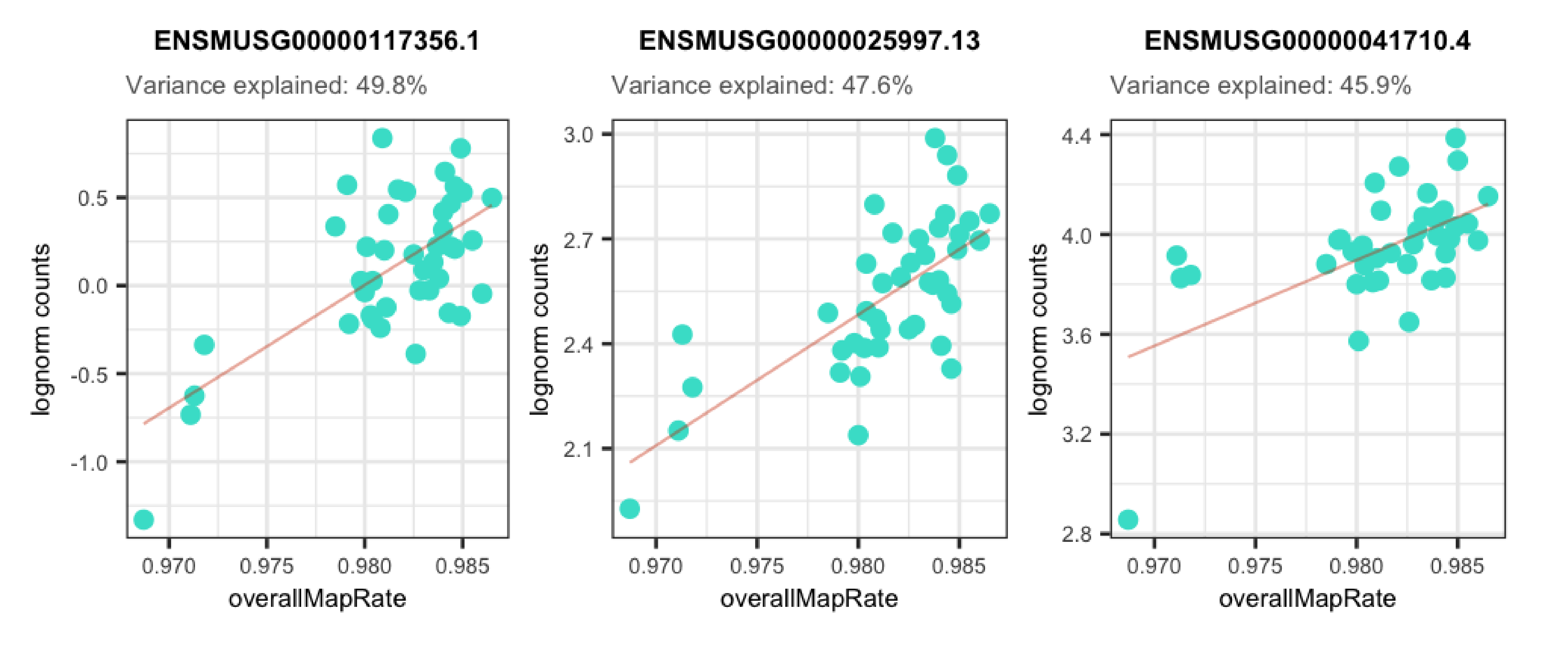
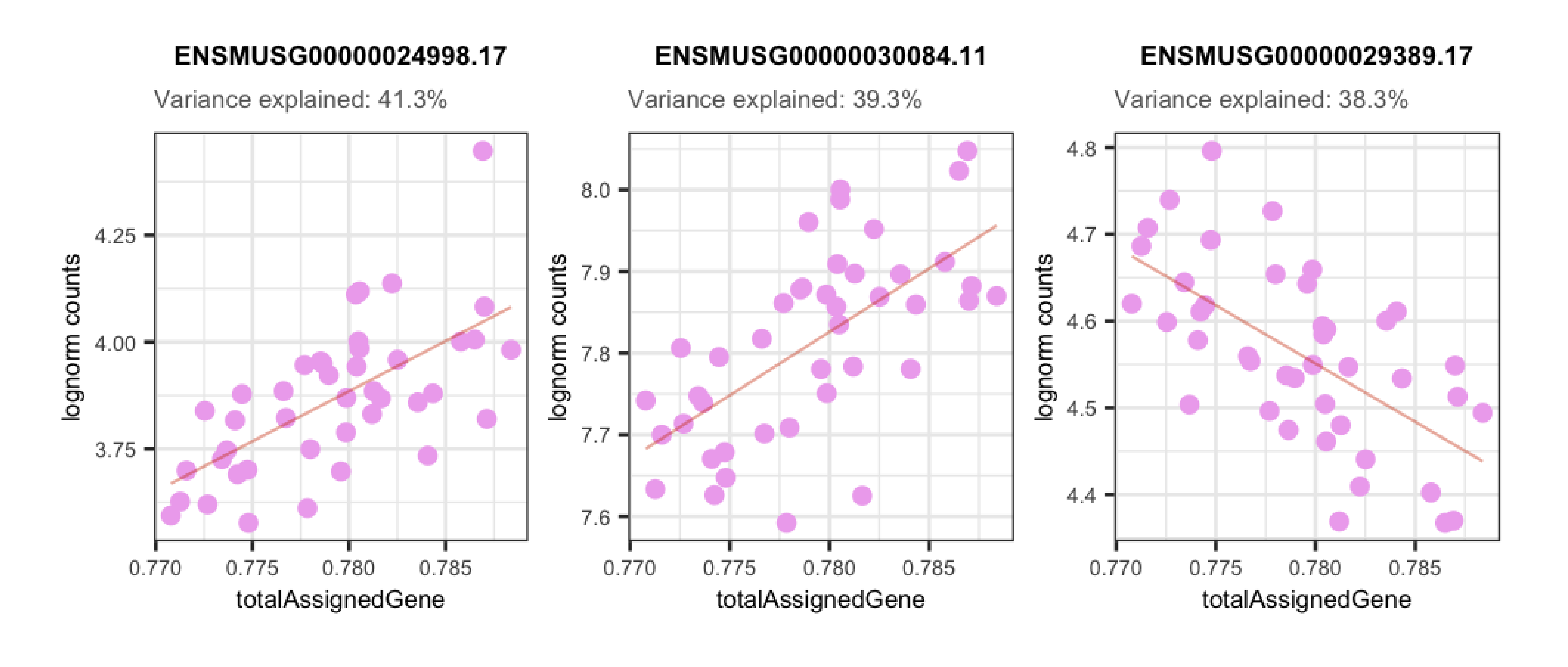
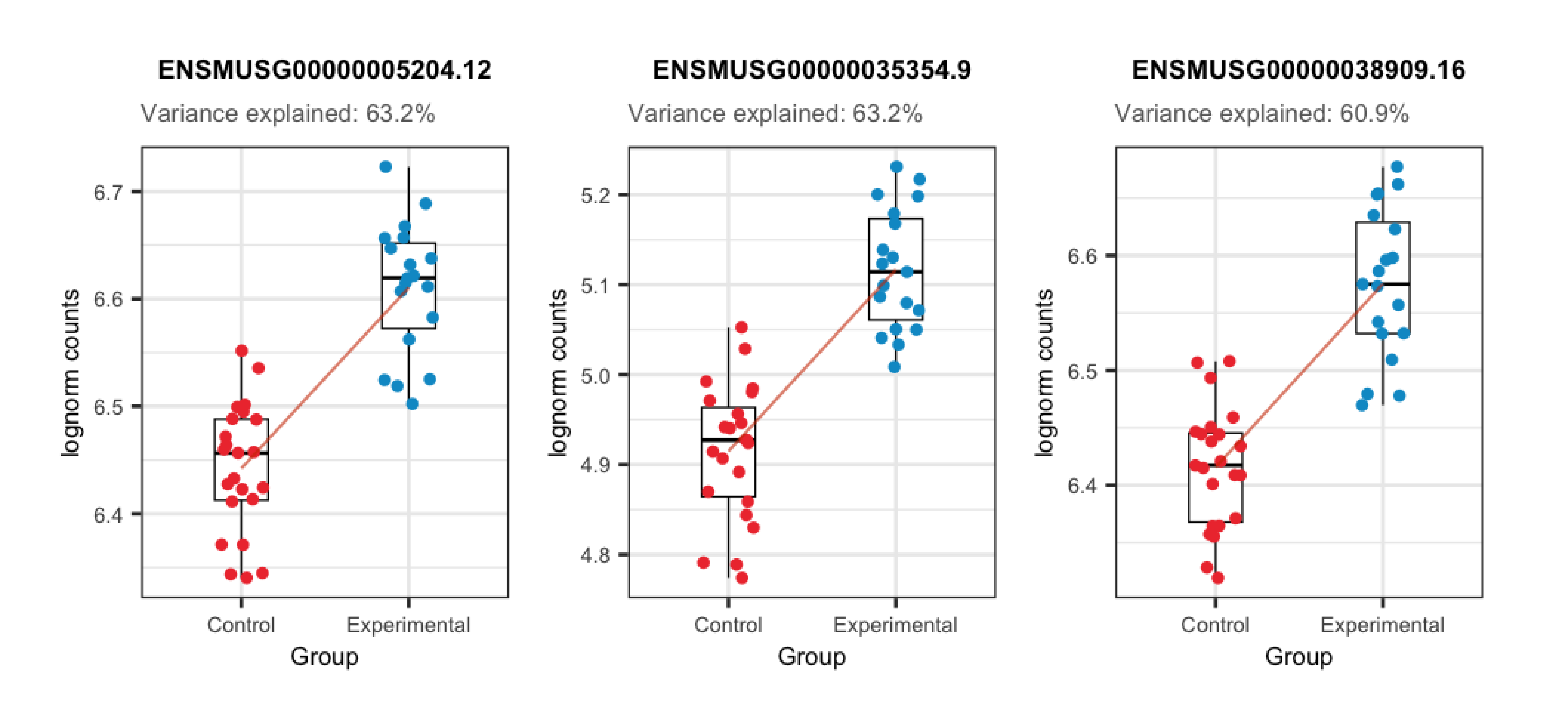
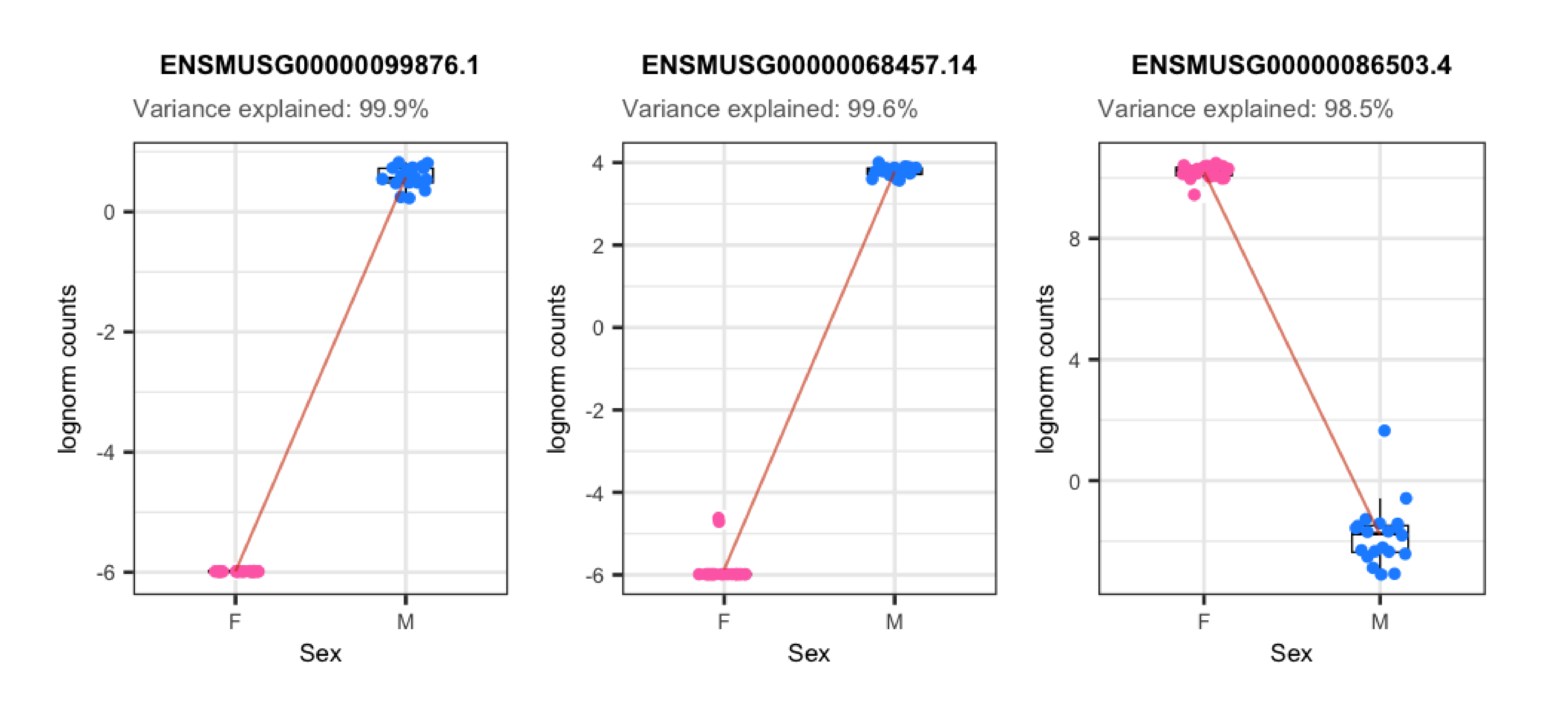
📝 Exercise 3: What % of variance does Group
explain for the gene ENSMUSG00000042348.10? Create the boxplots for its counts in control and experimental samples. Is it more likely that the gene is up-regulated or down-regulated?
📝 Exercise 4: Do the same for the gene ENSMUSG00000064372.1. What do you observe in terms of variance percentage and sample differences?
References
Hoffman, G. E., & Schadt, E. E. (2016). variancePartition: interpreting drivers of variation in complex gene expression studies.BMC bioinformatics, 17(1), 1-13.
Hoffman, G. (2022). variancePartition: Quantifying and interpreting drivers of variation in multilevel gene expression experiments.
van den Berg, S. M. (2022). Analysing data using linear models. Web site: https://bookdown.org/pingapang9/linear_models_bookdown/
Simoiu, C. & Savage, J. (2016). A bag of tips and tricks for dealing with scale issues. Web site: https://rpubs.com/jimsavage/scale_issues